我最近寫了一個小程式,幫助我自動化審核 LINE 社群(OpenChat)的加入申請,由於 LINE 社群並沒有提供 API 可以串接,而我的審核數量又非常大,因此我就想到了透過 OCR 辨識的方式來進行自動化。不過找了幾個免費的 OCR 套件,都沒有辦法很可靠的識別截圖中的文字,直到我嘗試了 Azure AI Vision 服務才眼睛為之一亮,這套產品的 Image analysis 功能品質極高,費用也極低,實際開發出東西後,我覺得這個服務真的非常值得推薦給大家。這篇文章我就帶大家用 .NET 8 簡單上手這個好用的雲端服務!
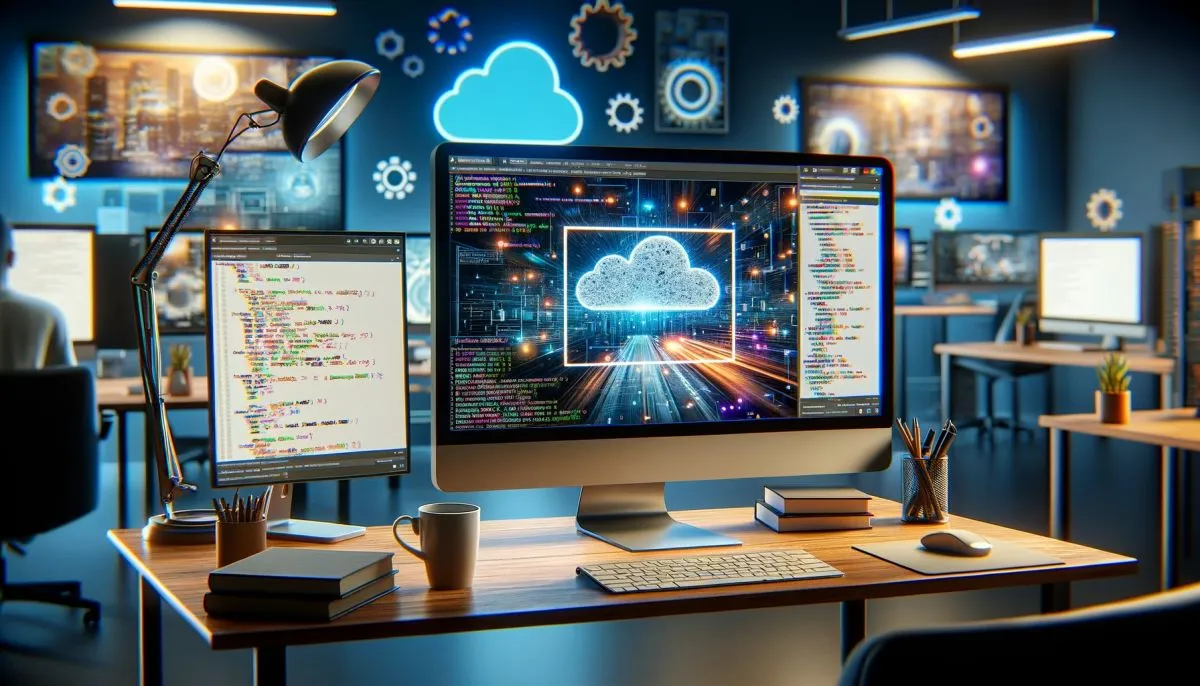
建立範例專案範本
-
建立 Console 專案
dotnet new console -n AzureAIVisionSDKDemo
cd AzureAIVisionSDKDemo
-
加入 Azure.AI.Vision.ImageAnalysis 套件
由於目前該套件還沒有穩定版本,因此安裝套件時要加上 --prerelease
參數
dotnet add package Azure.AI.Vision.ImageAnalysis --prerelease
-
加入 .gitignore
檔案
dotnet new gitignore
-
加入範例圖片 sample.jpg
請任意截圖,圖片上必須包含文字,並且將圖片放到專案根目錄中。
請將以下設定加入到 AzureAIVisionSDKDemo.csproj
檔案中:
<ItemGroup>
<Content Include="sample.jpg">
<CopyToOutputDirectory>PreserveNewest</CopyToOutputDirectory>
</Content>
</ItemGroup>
-
初始化專案完成,加入 Git 版控
git init
git add .
git commit -m "Initial commit"
撰寫主程式
我寫了一段非常簡單的範例程式,看程式應該蠻好理解的。
這段程式主要是透過 Azure AI Vision 來進行圖片文字辨識,並且將辨識結果顯示在 Console 畫面上。
using Azure;
using Azure.AI.Vision.Common;
using Azure.AI.Vision.ImageAnalysis;
var visionEndpoint = Environment.GetEnvironmentVariable("VISION_ENDPOINT");
var visionKey = Environment.GetEnvironmentVariable("VISION_KEY");
if (visionEndpoint is null || visionKey is null)
{
Console.WriteLine("The VISION_ENDPOINT or VISION_KEY environment variables are not set.");
return;
}
var serviceOptions = new VisionServiceOptions(visionEndpoint, new AzureKeyCredential(visionKey));
using var imageSource = VisionSource.FromFile("sample.jpg");
var analysisOptions = new ImageAnalysisOptions()
{
Features = ImageAnalysisFeature.Text,
Language = "en",
};
using var analyzer = new ImageAnalyzer(serviceOptions, imageSource, analysisOptions);
// 這行程式會把資料轉成 API 呼叫並且等待結果
var result = await analyzer.AnalyzeAsync();
if (result.Reason == ImageAnalysisResultReason.Analyzed)
{
if (result.Text != null)
{
Console.WriteLine($" Text:");
foreach (var line in result.Text.Lines)
{
string pointsToString = "{" + string.Join(',', line.BoundingPolygon.Select(pointsToString => pointsToString.ToString())) + "}";
Console.WriteLine($" Line: '{line.Content}', Bounding polygon {pointsToString}");
foreach (var word in line.Words)
{
pointsToString = "{" + string.Join(',', word.BoundingPolygon.Select(pointsToString => pointsToString.ToString())) + "}";
Console.WriteLine($" Word: '{word.Content}', Bounding polygon {pointsToString}, Confidence {word.Confidence:0.0000}");
}
}
}
}
我把這張圖的 OCR 識別結果整理在 https://github.com/doggy8088/AzureAIVisionSDKDemo 這個 Repo 中,大家可以上來看一下,OCR 識別的效果非常的好,而且會清楚標示出所有文字的座標,也因此我可以透過這些座標進行一些重要的自動化操作!👍
主程式的 result.Text.Lines
代表他識別出來的「每一行」文字,也就是說他是以「行」為單位進行識別的,然後才取出每一個「字」(Words
),無論是「行」或「字」都會提供完整的座標位置 (圖片的相對位置),以及最後還會給個「信心指數」(Confidence
),讓你可以判斷這個辨識結果的可信度。
發行注意事項
目前在發行有用到 Azure AI Vision SDK 的專案時,要注意一些地方,否則執行時會發生錯誤。
由於我的自動審核小程式必須登入 LINE 才能使用,我不想一直佔用我的滑鼠與鍵盤,所以我想把程式跑在 VM 裡面,然而一開始總是無法順利執行,會出現以下錯誤:

System.DllNotFoundException: Unable to load DLL 'Azure-AI-Vision-Native' or one of its dependencies: 找不到指定的模組。 (0x8007007E)
at Azure.AI.Common.Internal.Interop.Diagnostics.native.diagnostics_eventsource_logmessage_set_callback(IntPtr callback)
at Azure.AI.Common.Internal.Interop.Diagnostics.SetEventSourceMessageCallback(LogEventSourceMessageCallbackFunctionDelegate callback)
at Azure.AI.Vision.Common.Diagnostics.Logging.VisionEventSource..ctor()
at Azure.AI.Vision.Common.Diagnostics.Logging.VisionEventSource..cctor()
後來找了 Stack Overflow 的 DllNotFoundException: Unable to load DLL 'Azure-AI-Vision-Core' or one of its dependencies: The specified module could not be found. (0x8007007E) 這篇,無解。原始的回答只講對了一半,沒提到另一個重點,那就是還必須額外安裝 Microsoft Visual C++ Redistributable 套件!
以下是官方文件提及的基本要求:
- 如果要部署到 Windows 的話,僅支援 Windows 10 (或更高) 的版本
- 目前的 Azure AI Vision SDK 僅支援
x64
平台
- 目標電腦必須額外安裝 Microsoft Visual C++ Redistributable for Visual Studio 2015, 2017, 2019, and 2022
我把更完整的答案也貼到 Stack Overflow 了,有空的人可以幫我 Up 一下!
如果你要在 LINQPad 順利執行上述範例程式,還必須做出以下設定:
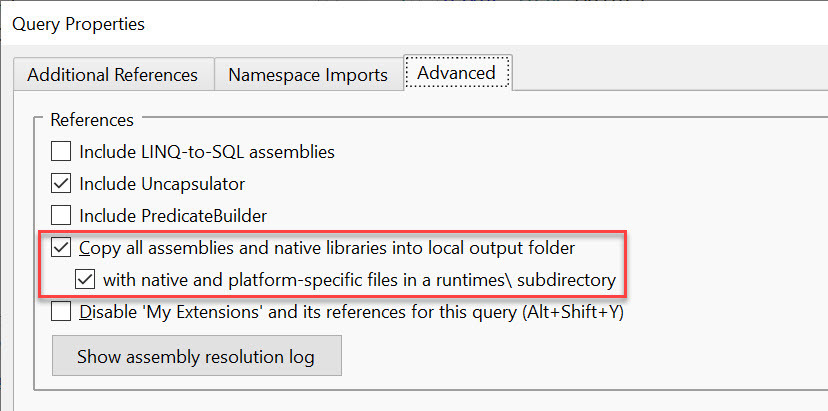
更完整的範例程式
Azure 官方有個 Azure-Samples 組織,裡面有超多範例程式,其中就有個 Azure-Samples/azure-ai-vision-sdk repo 整理了許多優質且完整的範例,算是學習 Azure AI Vision SDK 的一個不錯的起點!👍
如果要用 .NET 來開發的話,可以參考以下的範例程式:
相關連結